Rendering
This section will cover how rendering is achieved and how we'll use FnComponent's methods to create a to-do list.
HandleFn
type HandleFn func(context.Context) fncmp.FnComponent
fncmp.HandleFn accepts a context.Context and returns a FnComponent.
Always pass the context received by a HandleFn to fncmp.NewFn as it contains information needed by the client.
FnComponent
func fncmp.NewFn(context.Context, fncmp.Component) fncmp.FnComponent
fncmp.FnComponent is instantiated with a context.Context and a fncmp.Component. The context passed to NewFn should be the same context passed to the HandleFn.
Values may be stored and retrieved from the context as it's passed down to the FnComponent's children. A deeper look into the context will be covered in the next section.
func HandleMain(ctx context.Context) fncmp.FnComponent {
button := fncmp.HTML("<button>Click me</button>")
// pass the inherited context to the FnComponent
return fncmp.NewFn(ctx, button)
}
The DOM
If you aren't familiar with the term DOM, please refer to the MDN documentation.
For this tutorial we'll focus on the index.html file.
By default, components are rendered to the main
tag in the body of the HTML document, replacing it's inner content.
<!DOCTYPE html>
<html>
<head>
<title>My Project</title>
<script src="/static/fncmp.min.js"></script>
</head>
<body>
<main></main>
</body>
</html>
Selective rendering
There a eight methods available to FnComponent for rendering to specific elements in the DOM.
The default setting of a FnComponent is to replace the contents of the main
tag. This is equivalent to:
fncmp.NewFn(ctx, greeting).SwapTagInner("main")
- Tag
- Element
Parameter: tag string
- SwapTagInner (default) Swaps the inner HTML of a tag (eg. main) with the HTML rendered by the FnComponent
- SwapTagOuter Swaps the outer HTML of a tag with the HTML rendered by the FnComponent
- PrependTag Prepends the HTML of a tag with the HTML rendered by the FnComponent
- AppendTag Appends the HTML of a tag with the HTML rendered by the FnComponent
Parameter: id string
- SwapElementInner Swaps the inner HTML of an element by ID with the HTML rendered by the FnComponent
- SwapElementOuter Swaps the outer HTML of an element by ID with the HTML rendered by the FnComponent
- PrependElement Prepends the HTML of an element by ID with the HTML rendered by the FnComponent
- AppendElement Appends the HTML of an element by ID with the HTML rendered by the FnComponent
Example: AppendElement
For this demonstration, we'll update the index.html file instead of getting into templating and building components.
<!DOCTYPE html>
<html>
<head>
<title>My Project</title>
<script src="/static/fncmp.min.js"></script>
</head>
<body>
<main>
<div id="greetings"></div>
</main>
</body>
</html>
Instead of replacing the contents of the main
tag, we'll append the HTML to the div
with the id greetings
.
func handleClick(ctx context.Context) fncmp.FnComponent {
greeting := fncmp.HTML("<h1>Hello, world!</h1>")
fn := fncmp.NewFn(ctx, greeting)
return fn.AppendElement("greetings")
}
Now, instead of replacing the contents of the main
tag, the FnComponent will append to the main
tag so that the greeting div is not replaced.
func HandleMainFn(ctx context.Context) fncmp.FnComponent {
button := fncmp.HTML("<button>Click me</button>")
fn := fncmp.NewFn(ctx, button).WithEvents(handleClick, fncmp.OnClick)
return fn.AppendTag("main")
}
Clicking the button will append the greeting to the div
with the id greetings
.
Output:
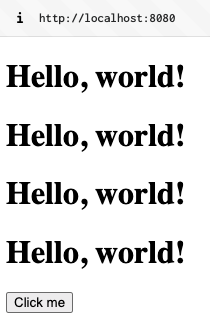